Access Activity class from View
Posted by Dimitri | Sep 26th, 2010 | Filed under Featured, Programming
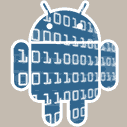
So, it begins! This is the first on this blog!
Let’s start with a Android Programming tip: how to access the Activity from an instantiated View object.
The first thing to do is consider if you really need to call an Activity from a View. Take some time to analyze your classes and their relationships. Probably, the variable/method you need to access doesn’t have to be a member of the Activity class. In that case, think about placing that variable/method inside the View or create a new class that has variables/methods which can be accessed by both Activity and View. Unless an Activity variable or method (e.g. Activity.finish() ) needs to be called from the View, there is no need to try to access the Activity from the current View.
But if you need to access a method/variable of the calling Activity from the View, here is how it’s done:
Assuming that you need to call the finish() method at Activity1 from View1:
((Activity1)getContext()).finish();
This is why the code above works: Activity1 is a child of the Activity class, which has Context as a parent. Due to polymorphism, a cast to Activity1 could be added to the method getContext() so it returns Activity1 instead of Context (Activity1 is a child of Context).
Using this method a View can access any public member from the Activity, not only the finish() method. This isn’t the best alternative, but sometimes, when programming a graphically intensive application like a game; it’s good to have access the Activity from the View.
You have no idea how long I have been looking for this simple solution. Thank you so much, I freaking love you.
Thanks! I’m glad you found what you were looking for.
Great simple article. I was also searching for this solution. I never thought to cast the Context object to my Activity object. Thanks for this.
Great article boss.Thank you.
Yesssssss!!!!!
Note that this approach does not always work, so be careful when doing this:
http://stackoverflow.com/questions/3901590/get-activity-object-while-in-view-context
According to Romain Guy (an Android framework engineer), the context of a view is not always guaranteed to be an activity.
Simple + Work = Great!
Fantastic! You’re the best!
Really good article! Thanx for sharing this ;)