Android: Changing the ‘back’ button behaviour
Posted by Dimitri | Dec 7th, 2010 | Filed under Programming
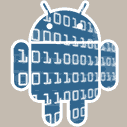
Sometimes, when programming Android applications, there is the need to assign another behavior for the ‘Back’ button that isn’t the default one. Although not recommended, there are some cases that changing the ‘Back’ button behavior is necessary, such as to avoid accidentally finishing the current Activity.
For example, a text editor, should confirm if the user really wants to quit without saving the current changes, or a game, that check if it is the player’s intention to forfeit the current game session.
So, to start changing the ‘Back’ button, behavior, you need to override the onKeyDown() method and than check if the desired button has been pressed:
//Don't forget this import import android.view.KeyEvent; public class YourActivity extends Activity { private View yourView; /** Called when the activity is first created. */ @Override public void onCreate(Bundle savedInstanceState) { //Initialization code //... //don't forget to make your view focusable. yourView.setFocusable(true); } //... //... //Override the onKeyDown method @Override public boolean onKeyDown(int keyCode, KeyEvent event) { //replaces the default 'Back' button action if(keyCode==KeyEvent.KEYCODE_BACK) { //do whatever you want the 'Back' button to do //as an example the 'Back' button is set to start a new Activity named 'NewActivity' this.startActivity(new Intent(YourActivity.this,NewActivity.class)); } return true; } }
As explained, this code overrides the OnKeyDown() method, that is called every time a key is pressed. The if statement inside this method checks if the ‘Back’ button has been pressed. If it does, the code inside the block will execute. As an example, at the above code, the ‘Back’ button starts a new Activity.
Don’t forget to set the return to true (as in line 32). This tells Android that the key press events had been handled and that they don’t need to propagate to the next Activity.
There’s also another way to do it, but your project has to be at least for Android 2.0. Instead of overriding the onKeyDown() method, use this other one instead:
@Override public void onBackPressed() { //do whatever you want the 'Back' button to do //as an example the 'Back' button is set to start a new Activity named 'NewActivity' this.startActivity(new Intent(YourActivity.this,NewActivity.class)); return; }
Be the first to leave a comment!