Android: reading float values from a XML file
Posted by Dimitri | Jun 3rd, 2011 | Filed under Programming
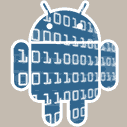
When programming an Android app, data such as integers, drawables and strings can be loaded from XML files through the Resources class, using their automatically generated IDs. But a float can’t be placed in these XML files, since the Resources class won’t generate IDs for it. This post will focus on explaining how to load a float, and some other values from a customized XML file. All code featured in this tutorial is available at the end of the post.
It’s worth mentioning that this example uses the XmlResourceParser class (a XML pull parser) to read the contents from a XML file. This is just one of the three available methods of retrieving the contents of a XML file in Android. That being said, the first thing we are going to need is the XML file. However, instead of creating it inside the res/values folder, create it at the res/xml folder. To create it using Eclipse, right click the res folder and select New->Folder. Repeat this process to create the XML file, although this time, select just ‘File‘ from the drop down menu. At the next dialog, give it a name, like custom.xml, and don’t forget to add the .xml extension.
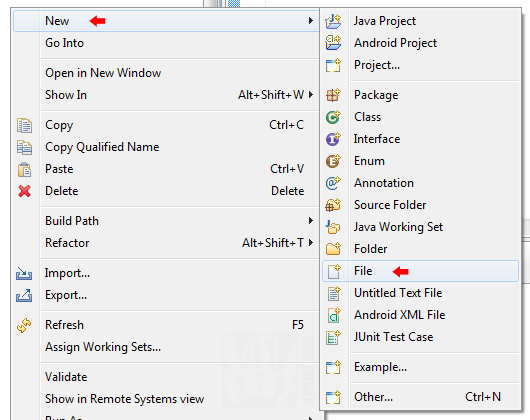
Create the file inside the 'xml' folder, as shown in the picture.
Now, it’s time to add some data to the XML file. The rest of this example will retrieve the contents from this file:
<tutorial id="level" difficulty="1.5" enemies="3">The tutorial level. </tutorial> <level1 id="level" difficulty="2.6" enemies="10">The first level. </level1>
And that’s it for the XML. The following code is an Activity, which calls a method that reads the contents from the above XML, using the XmlResourceParser class:
package fortyonepost.com.cx; import java.io.IOException; import org.xmlpull.v1.XmlPullParser; import org.xmlpull.v1.XmlPullParserException; import teste.com.teste.R; import android.app.Activity; import android.content.res.Resources; import android.content.res.XmlResourceParser; import android.os.Bundle; import android.util.Log; import android.widget.Toast; public class CustomXML extends Activity { //this object will store a reference to the Activity's resources private Resources resources; //this integer will store the XMLResourceParser events private int event; //a boolean that will tell if it's the tag we are looking for private boolean isCorrectTag = false; /** Called when the activity is first created. */ @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.main); //get the application's resources resources = this.getResources(); try { GetAttributes("tutorial"); GetAttributes("level1"); } catch (XmlPullParserException e) { //Display a toast message. Toast.makeText(this, "Could not retrieve the current parser event", Toast.LENGTH_SHORT); } catch (IOException e) { //Display a toast message. Toast.makeText(this, "Could not read XML file", Toast.LENGTH_SHORT); } } public void GetAttributes(String tagName) throws XmlPullParserException, IOException { //get a XmlResourceParser object to read contents of the 'custom.xml' file XmlResourceParser parser = resources.getXml(R.xml.custom); //get the initial parser event event = parser.getEventType(); //while haven't reached the end of the XML file while (event != XmlPullParser.END_DOCUMENT) { //get the type of the event event = parser.getEventType(); //if parser finds a new tag and its name equals 'tagName' if (event == XmlPullParser.START_TAG && parser.getName().contentEquals(tagName)) { //print the attribute values at LogCat //print the ID Log.i("CustomXML", "id:" + parser.getIdAttribute()); //print the difficulty float difficulty = parser.getAttributeFloatValue(null, "difficulty", 0.0f); Log.i("CustomXML", "difficulty:" + Float.toString(difficulty)); //print the number of enemies int enemies = parser.getAttributeIntValue(null, "enemies", 0); Log.i("CustomXML", "enemies:" + Integer.toString(enemies)); //Found the tag, set the boolean to true isCorrectTag = true; } else if (event == XmlPullParser.TEXT && isCorrectTag) { //Print the tag's content Log.i("CustomXML", parser.getText()); //set isCorrectTag to false; isCorrectTag = false; //break the loop, we already found what we were looking for break; } //go to the next tag parser.next(); } //Release resources associated with the parser parser.close(); } }
Right at the beginning at the code, three different private members are declared. The first one is an object of the Resources class, which will store a reference to the application’s resources (line 20). The second one is a an Integer named event, that will keep a reference to the XML parser events (line 22). The third one is a boolean, that flags if the parser has reached the desired tag (line 24).
Next, the onCreate() method, a reference to the app’s resources is obtained and stored at the resources variable (line 34).
Let’s jump straight to line 53, at the GetAttributes() method. In short, it will take a String as a parameter and use it to compare with the tags that the XML parser pulls from the XML file. After the tag has been found, it will print the attributes of these tag in LogCat and change the isCorrectTag variable to true, also printing the contents inside the tag when the parser reaches it.
For that, line 56 declares and initializes the XmlResourceParser object, conveniently named parser. Then, the initial event of the parser is stored at the event variable (line 59).
A while loop is started at line 62, and its is set to stop only when the parser reaches the end of the XML file. Inside it, the parser event is again obtained, since its type will be checked inside the loop (line 65). The first if statement in the loop checks if the parser reached a tag that has the same name as the tagName passed as a parameter to the method (line 68). On a side note, it’s possible to check for other events. click here for a list of the possible ones.
Now back to the code, lines 69 through 84 prints the attributes at LogCat. The first one is the id; obtained by a the getIdAttribute() method (line 72). The id attribute is the only one that has a dedicated method to retrieve it, so it’s wise to use it in the XML file.
The other attributes, such as the difficulty and enemies are obtained by the getAttribute<Type>Value() methods, where <type> can be replaced by Float, Int, UnsignedInt, List and String, depending on how you want the attribute to be interpreted. These methods returns the attribute’s value through their name, passed as the second parameter. The third parameter is the default value that is used just in case the attribute haven’t been found.
Alternatively, it is possible to get the attributes using a different version of those methods, that takes two parameters: the first one is an index and the second one is the default value. Returning the attribute by the index number is faster than returning it by the name, as an example, line 75 could be written as:
//the 'difficulty' is the second attribute - index 1 parser.getAttributeFloatValue(1, 0.0f);
The last line of code (line 83) inside this if statement sets the isCorrectTag boolean to true. This variable is used as part of the next expression checked by the else if block (line 85). The code inside this block will only execute if the parser has reached the tag’s content and the isCorrectTag boolean is true. It prints the contents of the tag at LogCat and breaks the while loop (lines 88 and 90). Outside the two if statements, the parser.next() (line 95) retrieves the next parser event. Line 98 releases the resources associated with the parser.
Finally, back to the onCreate() method, the GetAttributes() is called twice and since it throws two exceptions, the method calls are placed inside a try/catch block (lines 36 through 50).
Hope this serves as an introduction to retrieving float and other types of values from custom XML files. For the code featured in this tutorial, download the compressed Eclipse project below.