Unity: GUI Toggle Pair
Posted by Dimitri | Apr 21st, 2013 | Filed under Programming
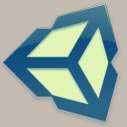
This new (and brief) Unity programming post explains how to create a pair of GUI.Toggles that modifies the value of a single boolean. This means that, when one toggle is selected, the other one should be automatically set to unselected. This is best explained with an example, which can downloaded at the end of the post.
In this example, the value of the boolean day will be set using two toggles. When the toggle “Day” is selected, the “Night” toggle should be unselected. This must also work on the other way around, like this:
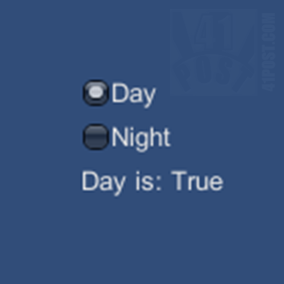
In this example, the day boolean is set to true.
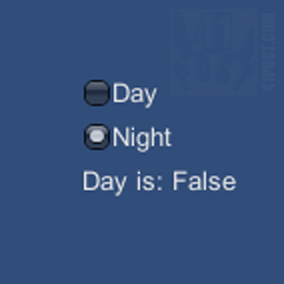
At the above image, the day boolean is set to false.
To make the day boolean value be set by both toggles, one toggle should return the inverse of the value of the boolean the other toggle has just set. Here’s the code:
using UnityEngine; using System.Collections; public class TogglePair : MonoBehaviour { //Create and initialize a boolean to be toggled private bool day = true; void OnGUI() { //Render the toggle normally this.day = GUILayout.Toggle(this.day,"Day"); /* Invert the boolean value and return the inverse of * what has been set on the toggle.*/ this.day = !GUILayout.Toggle(!this.day,"Night"); //Render the selected value. GUILayout.Label("Day is: " + this.day.ToString()); } }
The code should be self explanatory. Basically, the boolean day is created and initialized (line 7). Then, the first toggle is rendered, just as described on the official documentation (line 12). The second toggle, the one labeled “Night”, is rendered just as the previous one, but with a couple of differences.
The inverted (note the ! sign) value of the day boolean is passed as the first parameter to the GUILayout.Toggle() method. This makes the toggle render the correct state, which is the inverse of the day variable value. Additionally, the value of the boolean returned by the GUILayout.Toggle() method is inverted (again, notice the ! sign). It makes the GUILayout.Toggle() method return the correct boolean value, which is stored in the day boolean. In other words, when the “Night” toggle is selected, the day should be set to false.
That’s it! Both JavaScript and C# versions of this script can be found at the example project:
Downloads
Be the first to leave a comment!